Stop Blocking Your Threads: How (and When) to Use ConfigureAwait(false)
Because your API deserves to run faster than a snail on sedatives.
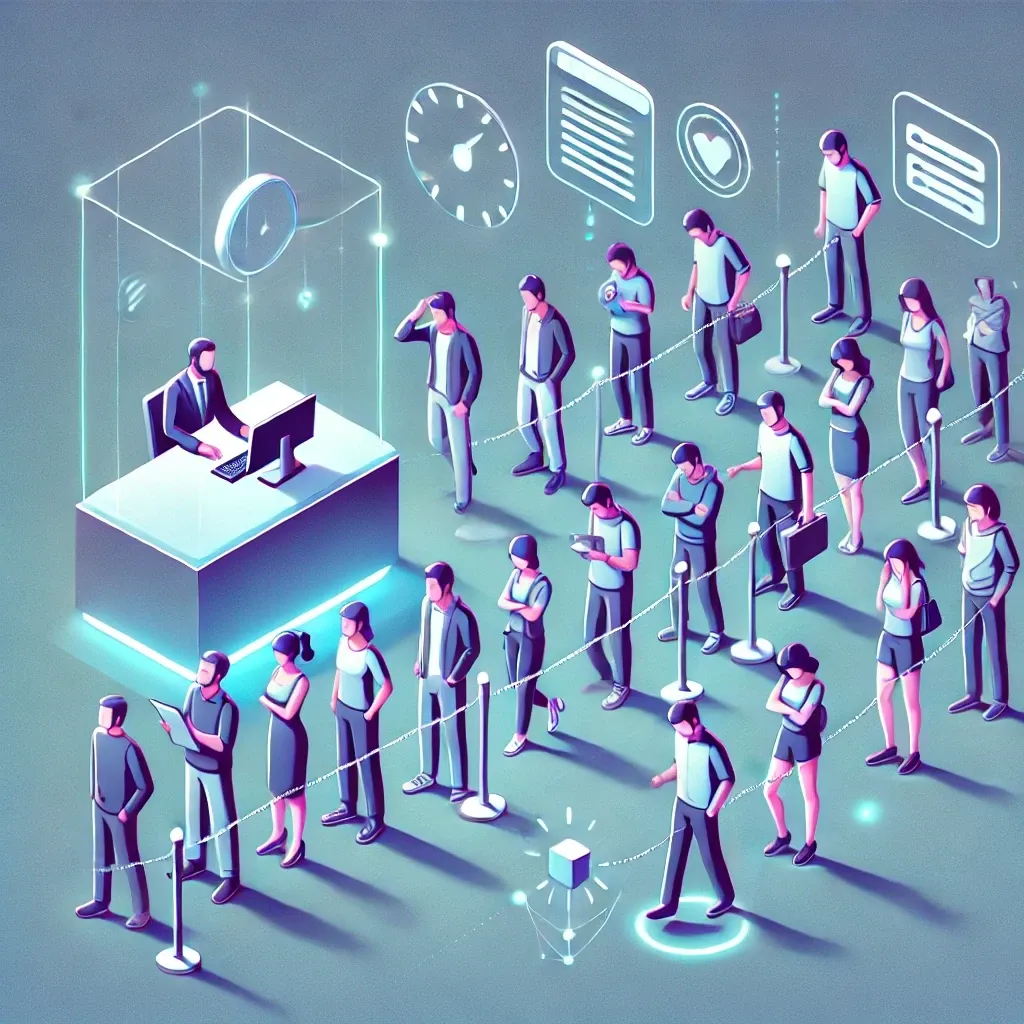
Why Should You Care?
You're writing async code like a good little developer, but something feels... off. Your API is sluggish under load, or maybe it’s mysteriously locking up in production. And worst of all, someone just told you to slap ConfigureAwait(false)
on everything without explaining why.
Well, I’m about to blow your mind, because this one tiny method call might be the performance boost your .NET app needs. It might also be the cause of your next headache, so read carefully!
What Does ConfigureAwait(false)
Actually Do?
When you await
something in .NET, the default behaviour is to capture the SynchronizationContext
or TaskScheduler
and return execution to the original context once the awaited task completes.
ConfigureAwait(false)
tells .NET: “I don’t care what thread I resume on. Just get it done.”
Why does this matter?
- In UI applications (WinForms/WPF), you must return to the UI thread to update the UI. That’s why .NET keeps track of what thread you're on.
- In ASP.NET Core, there is no UI thread. There’s no need to jump back to a specific thread—so keeping that context around is just wasted effort.
🚀 TL;DR: ConfigureAwait(false)
reduces thread switching overhead, making your API faster and more scalable.
When You Should Use ConfigureAwait(false)
✅ Use It in Library Code
If you’re writing a reusable .NET library, always use ConfigureAwait(false)
. You have no idea where your code will run—web app, desktop app, console app—so don’t assume it needs a specific context.
public async Task<string> FetchDataAsync()
{
using var client = new HttpClient();
return await client.GetStringAsync("https://ciarancodes.com").ConfigureAwait(false);
}
🔍 Why? Avoids unnecessary thread switching in API scenarios while still working fine in UI apps.
✅ Use It for I/O-Bound Operations
If you’re calling a database, making an HTTP request, or reading a file, none of these need to return to the same thread.
public async Task<List<Product>> GetProductsAsync()
{
using var db = new MyDbContext();
return await db.Products.ToListAsync().ConfigureAwait(false);
}
🚀 Benefit: Your API thread pool won’t get clogged up waiting on database queries.
✅ Use It in High-Throughput Web APIs
Your ASP.NET Core API doesn’t care what thread it's using. It just wants to finish processing requests as fast as possible.
public async Task<IActionResult> GetData()
{
var data = await FetchDataAsync().ConfigureAwait(false);
return Ok(data);
}
🏎️ Faster Execution: More requests handled per second, because fewer threads are blocked.
When You Should NOT Use ConfigureAwait(false)
❌ In UI Code (WinForms, WPF)
If you need to update UI elements, you must stay on the main thread.
🚨 Bad: UI Update Will Crash
public async Task UpdateLabelAsync()
{
label.Text = "Loading...";
await Task.Delay(1000).ConfigureAwait(false);
label.Text = "Done!"; // ❌ CRASH: Not on UI thread!
}
✅ Fix: Omit ConfigureAwait(false)
(or use true
, which is the default)
public async Task UpdateLabelAsync()
{
label.Text = "Loading...";
await Task.Delay(1000); // Will continue on the UI thread
label.Text = "Done!";
}
Does ConfigureAwait(false)
Improve Performance?
✅ Yes—but only in the right places. Let’s break it down:
- ASP.NET Core: No
SynchronizationContext
, soConfigureAwait(false)
mostly saves minor task-scheduling overhead. Good practice, but not a game-changer. - Console Apps & Services: Helps in background processing, reduces potential deadlocks.
- UI Apps (WinForms/WPF): Do NOT use it if you need to update the UI.
Common Misconceptions
❌ "I should use ConfigureAwait(false)
everywhere!"
- No. Only use it where context-switching doesn’t matter.
❌ "It makes everything async!"
- Nope. It just stops forcing your task to return to the original thread.
❌ "ASP.NET Core requires ConfigureAwait(false)
!"
- Not true. ASP.NET Core has no
SynchronizationContext
, so it’s technically unnecessary. But adding it still prevents unnecessary scheduling overhead.
Final Verdict: Should You Use ConfigureAwait(false)
?
✅ Yes - When you don’t need to return to the same thread (e.g., database calls, APIs, background tasks). ❌ No - If you’re in a UI app and need to touch UI elements after an await
. 🤔 Maybe - If you’re in ASP.NET Core. It won’t break anything, but the performance gain is minor.
Summary (For the TL;DR Crowd)
✔️ ConfigureAwait(false)
reduces thread switching overhead by avoiding default context capture.
✔️ Use it in library code, APIs, and async I/O operations (database queries, HTTP calls, file I/O).
❌ Don’t use it in UI code if you need to update the UI thread.
✔️ ASP.NET Core doesn’t need it, but using it still provides slight optimizations.
Final Thought: One Word, Big Impact
Adding .ConfigureAwait(false)
is like switching to SSDs from HDDs: the difference isn’t always massive, but in high-performance scenarios, every little bit helps.
Now go forth, use it wisely, and stop blocking your threads like a rookie. 🚀