Beyond the Interview: Data Structures (#3 Stacks & Queues)
Stacks go LIFO, queues go FIFO, and somewhere in the chaos, your job scheduler just rage-quit. Let’s cut the fluff and decode the real-world uses (and abuses) of these core data structures.
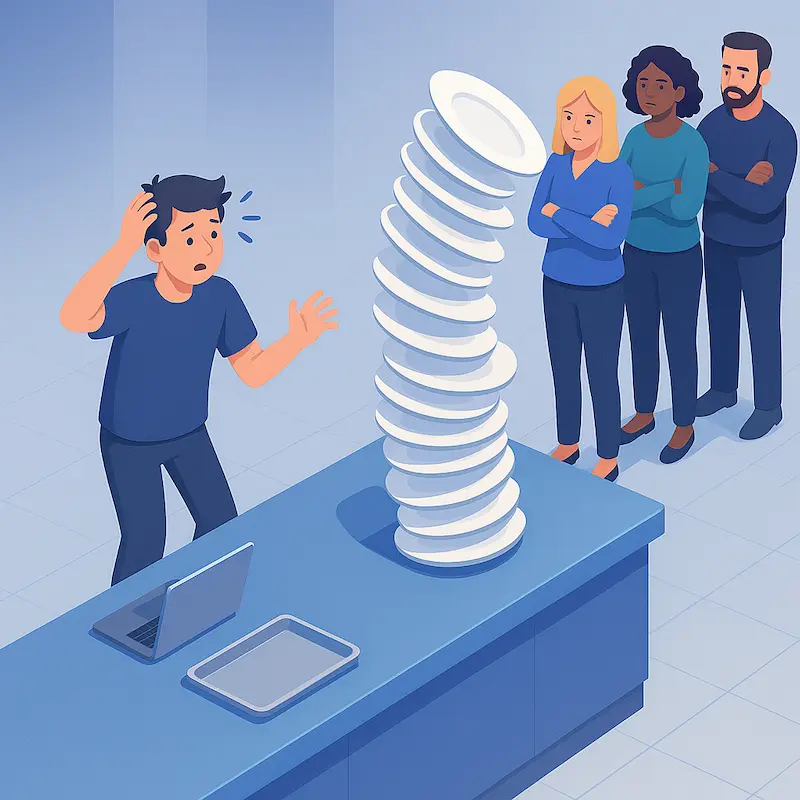
About This Series
We’ve all been there—grinding LeetCode, memorizing tree traversals, and pretending to care about Red-Black Trees because some Big Tech interviewer insists they’re “fundamental.”
But here’s the reality: 99% of the time, you’re just using Lists, Arrays, and HashMaps—and that’s fine.
Read more
So why bother with the rest? Because sometimes the default isn’t the best choice.
- Maybe a different data structure completely revolutionizes your system.
- Maybe it opens up new possibilities you hadn’t considered.
- Maybe it’s the key to removing that one persistent bottleneck that refuses to budge no matter how many CPU cores you throw at it.
Some data structures solve real-world problems, while others exist purely to torture CS students. In this series, we’ll cut through the nonsense—breaking down:
✅ When data structures actually matter
✅ What trade-offs they introduce
✅ Where they fit into practical software development
No fluff. No pointless academic exercises. Just real-world insights, benchmarks, and maybe a few laughs along the way.
Bonus: In your next tech interview, you’ll be able to talk about the pros and cons of Bloom Filters and Suffix Trees as easily as reversing an array—and that will make you stand out.
I mention some internal workings related to performance (stack vs heap, garbage collection, etc). If you’re not up to speed, check out Dig Deeper: Memory Management in .NET].
Explore This Series
Arrays & Lists | Linked Lists | Stacks & Queues | Hash Tables & Bloom Filters
🥞 Stacks & Queues – LIFO, FIFO, and WTF-IFO?
The Line Starts Here
You're not managing Hogwarts students or parsing tiny expressions—you're optimizing job queues, navigating call stacks, or juggling background tasks.
TL;DR
- Stack = LIFO (Last In, First Out). Think pancakes.
- Queue = FIFO (First In, First Out). Think British people waiting for tea.
- And yes, they’ve got weird cousins. Let’s meet them.
🥞 Section 1: Stacks – The Pancake Pile of Doom
1.1 What Is a Stack?
If you’ve ever hit a stack overflow exception, congrats—you’ve already met this beast. A Stack is a data structure where the last thing you put in is the first to come out.
LIFO: Last In, First Out. Like putting plates on a shelf, then pulling the top one off because it’s the cleanest. Then crying when the whole stack collapses.
Visuals you’ve definitely used a stack in:
- The call stack when debugging that one recursive method that should have stopped.
- Pressing
UP
in your terminal 300 times trying to find thels
from hours ago. - Undo/redo in a text editor (aka "oops buffers").
- Parsing expressions and math equations like a wizard.
1.2 Implementations
- Arrays: Fast. Simple. Fixed-size unless dynamic. Like IKEA shelves—great until you run out of space.
- Linked Lists: Slower but flexible. Can grow forever, like your tab count.
Basic operations:
Push
: Add an element.Pop
: Remove the top element.Peek
: Look at the top without messing it up (like your last clean hoodie before a Zoom call).
1.3 Real-World Use Cases
- Function call management (your runtime's backstage crew).
- Undo/redo systems in text editors, graphic tools, or your VS Code plugin obsession.
- Syntax and expression parsing in compilers, calculators, and that custom scripting engine your manager swore was “super quick to build.”
1.4 ⚠️ Gotchas That Will Trip You Up
- Stack overflow (no, not the site): Blow the stack with uncontrolled recursion and you’re in runtime hell.
- Mutability in threads: Stacks aren’t always thread-safe. A rogue pop in one thread can mess everything up.
- Recursion limits: Languages like C# and Java cap your recursive dreams. Watch your depth, Frodo.
🇬🇧 Section 2: Queues – The World's Most British Data Structure
2.1 What Is a Queue?
A Queue is a structure where the first thing in is the first to come out.
FIFO: First In, First Out. Just like waiting your turn at a chippy. Not like trying to get off a Ryanair flight.
Common uses:
- Task scheduling
- Print queues (yes, they still exist)
- Message buffering
- People pretending they’ve implemented a job system from scratch
2.2 Implementations
- Array-based: Basic. But unless you shift every element (slow), you’ll waste space. Or use circular indexing. Fancy.
- Linked list: Keep two pointers—
head
andtail
. If one’s wrong, welcome to NullReferenceException Land™.
2.3 Circular Queues
Why?
- In array-based queues, emptying elements still wastes space unless you loop around.
- Circular queues solve this using modulo math and the wrap-around fairy. 🧚
Use them when:
- You need bounded memory.
- You want to pretend you’re writing firmware for an 80s space probe.
2.4 Real-World Use Cases
- Background task queues (e.g.,
IHostedService
in .NET Core) - Keyboard input buffers in games and embedded systems
- Network request rate limiting (aka “stop spamming the API”)
2.5 ⚠️ Gotchas
- Off-by-one errors: Welcome to classic queue hell.
- Null pointers: One wrong move in a linked list queue and everything falls apart.
- Memory leaks: Not dequeuing = eternal bloat. Congrats, your app is now Chrome.
👑 Section 3: Priority Queues – When Every Task Thinks It's Special
3.1 What’s a Priority Queue?
A queue where elements are dequeued based on priority, not order. Like corporate emails—urgent gets read, the rest rot in "Unread" for eternity.
Usually implemented as a heap—a binary tree that knows who’s boss.
3.2 Real Use Cases
- OS-level task schedulers
- AI pathfinding (Dijkstra, A*, or “don’t walk into lava again”)
- Load balancers and print queues that never seem to print your job
3.3 Implementation Note
Sure, you can sort an array each time you enqueue. If you hate performance.
Use:
- .NETs
PriorityQueue<TElement, TPriority>
(added in .NET 6, finally). - A heap if you’re feeling algorithmic.
🔁 Section 4: Deques – The Switch-Hitter of Queues
4.1 What’s a Deque?
Double-Ended Queue—you can push and pop from both ends. Great for when your structure needs to commit to absolutely nothing.
Use it for:
- Sliding window problems (common in signal processing or "I saw this once in Leetcode").
- Fancy caching strategies.
4.2 Use Cases That Don’t Sound Boring
- Browser history: Back and forward, unless you're in Safari.
- Snake game mechanics: Add head, remove tail. Classic.
- LRU caches: Combine with a hash map and impress your interviewer.
4.3 ⚠️ Gotchas
- Criminally underused.
- Often misunderstood.
- Sometimes overkill when a simple queue does the job. But when it fits, it slaps.
🧮 Comparing the Lot
Structure | Access Pattern | Typical Use Case |
---|---|---|
Stack | LIFO (Last In, First Out) | Call stacks, undo/redo |
Queue | FIFO (First In, First Out) | Task queues, buffers |
Circular Queue | FIFO with wrap-around | Bounded systems, embedded apps |
Priority Queue | Dequeue by priority | Schedulers, A* search, load balancing |
Deque | Double-ended | LRU cache, sliding window problems |
Use the right tool. Not every job needs a custom-built golden hammer. Sometimes, a rusty screwdriver will do—especially if it’s O(1) time complexity.
🧠 Section 5: Interview ≠ Real Life (Again)
In interviews:
- You explain the Big-O like a TED Talk.
- You write out a priority queue from scratch using your tears.
In real life:
- You use a library.
- You prioritize reliability over academic purity.
- You don’t reinvent Redis. (Please. Just don’t.)
Remember:
- Know how it works.
- Know when to reach for it.
- Know when to walk away and just Google “best .NET queue implementation for async processing.”
🧁 That’s a Wrap
Stacks and Queues might look basic, but they're the sugar and flour of your software cake. Get them wrong, and the whole thing collapses. Get them right, and you’ve got elegant, efficient, maintainable code—and probably fewer midnight crashes.
Just remember: the real stack overflow… is the friends we made along the way. 😅